Authentication and Authorization with AWS - Federated Access
Nov 21, 2021
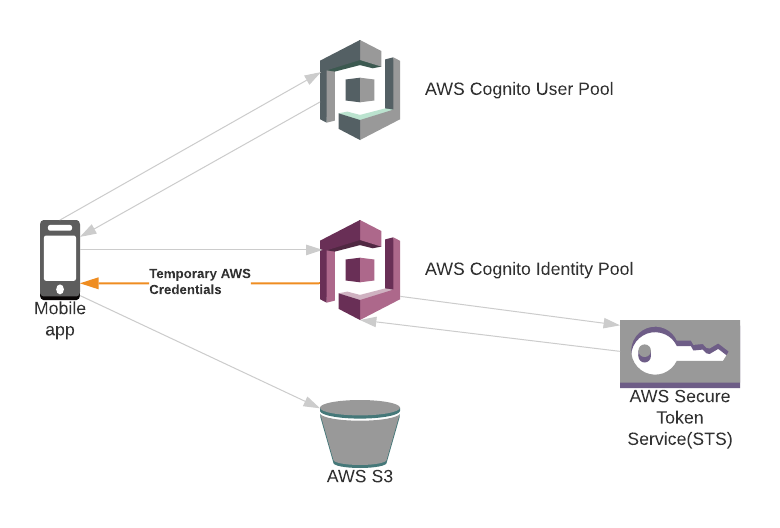
AWS Cognito Identity Pools provide authenticated users access to AWS resources. AWS Cognito can authenticate users with several methods, including Cognito User Pools. This post will examine how an authenticated user can be authorized to access an AWS S3 bucket.
Create an Identity Pool linked to the User Pool
Before creating the Identity pool, note the User Pool ID and App client ID. They will be needed when creating the Identity Pool.
Roles for Authenticated and Unauthenticated Users
Two roles must be present or created during the process - one for authenticated users and another for unauthenticated users. Unauthenticated users can be provided tokens from the Identity Pool if the application allows visitors to take action. When using existing rules, ensure a trust relationship with cognito-identity.amazonaws.com
, with an equality condition between cognito-identity.amazonaws.com:aud
and the Identity Pool ID.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"Federated": "cognito-identity.amazonaws.com"
},
"Action": "sts:AssumeRoleWithWebIdentity",
"Condition": {
"StringEquals": {
"cognito-identity.amazonaws.com:aud": "<Identity Pool ID>"
},
"ForAnyValue:StringLike": {
"cognito-identity.amazonaws.com:amr": "authenticated"
}
}
}
]
}
Federated access to S3 bucket
We will modify the listener from the User Pool sign-in process to use the id_token
on successful login to obtain temporary credentials and access the S3 bucket.
import { fromCognitoIdentityPool } from '@aws-sdk/credential-providers'
import { S3 } from '@aws-sdk/client-s3'
// app is an express() instance. Code omitted for brevity.
app.get('/sign-in', async (req, res) => {
console.log(req.query)
try {
const tokens = await requestToken({
code: req.query.code,
authSecret,
hostname: `${domain}.auth.${region}.amazoncognito.com`,
redirect_uri: 'http://localhost:9000/sign-in'
}) // requestToken fetches tokens from /oauth2/token endpoint
const loginMap = {}
// Resolving the id_token is a hack, actual code should use the AWS library
loginMap[`cognito-idp.${region}.amazonaws.com/${userPoolId}`] = () => Promise.resolve(tokens['id_token'])
const credentialProvider = fromCognitoIdentityPool({
identityPoolId,
logins: loginMap,
clientConfig: { region }
})
try {
const credentials = await credentialProvider()
console.log(credentials) // temporary credentials
const s3 = new S3({ credentials, region: 'us-east-1' })
const objects = await s3.listObjectsV2({Bucket: 'sayantam-hailstorm-ci'})
console.log(objects.Contents) // lists the bucket contents
res.sendStatus(200)
} catch (error) {
console.error(error)
throw error
}
} catch (_error) {
res.sendStatus(500)
}
})
The temporary credentials have the following structure:
{
identityId: 'ap-south-1:3383ad41-e061-8645-91b8-1a179ea2f5f7',
accessKeyId: 'ASI5ZGSSED',
secretAccessKey: 'BVUo0xL33juUL53V4QJotG1nqpD+C9vu/OK',
sessionToken: 'IQoJb3JpZ28ru+SR2w==',
expiration: 2021-12-12T17:07:32.000Z
}
Authorization use cases
This post concludes the federated access workflow. The following post will utilize the workflow for authorizing API gateways.
Related Posts
Authentication and Authorization with AWS - API Gateway
Dec 29 2021
AWS Cognito User Pools and Federated Identities can be used to authorize API gateway requests.
Authentication and Authorization with AWS - Cognito SAML Integration
Nov 14 2021
AWS Cognito integrates with a corporate identity provider such as Active Directory (AD) using SAML.
Authentication and Authorization with AWS - About IAM
Sep 12 2021
Amazon Web Services (AWS) references a dizzying number of concepts, resources, patterns, and best practices to provide a fully managed…
Authentication and Authorization with AWS - Cognito Sign-up and Sign-in
Oct 17 2021
Amazon Web Services (AWS) provides Cognito to delegate authentication and authorization out of applications completely.